इस पोस्ट में हम प्रोग्राम के रूप में स्विफ्ट में Spotify होम स्क्रीन लेआउट को फिर से बनाने की कोशिश करेंगे। प्रोग्रामेटिक रूप से क्यों? मुझे लगता है कि यह जानना हमेशा अच्छा होता है कि चीजों को अलग-अलग तरीकों से कैसे बनाया जाता है, और मुझे प्रोग्राम को प्रोग्रामेटिक रूप से करने के लिए कोड लिखना पसंद है। यदि आप टीम के साथ काम कर रहे हैं या संस्करण नियंत्रण का उपयोग कर रहे हैं तो ये कौशल विशेष रूप से सहायक होते हैं।

यह Spotify के मोबाइल ऐप की वास्तविक होम स्क्रीन है। तो इस प्रकार के लेआउट को प्राप्त करने के लिए, हम UICollectionView
. का उपयोग करेंगे , और हम TabBarController
. का उपयोग कर सकते हैं साथ ही टैब नेविगेटर बनाने के लिए।
मूल आवश्यकता :पहले सुनिश्चित करें कि आपके पास Xcode +10 स्थापित है और +4 तेज़ है।
आइए Xcode का उपयोग करके एक नया Xcode प्रोजेक्ट बनाकर शुरू करें:
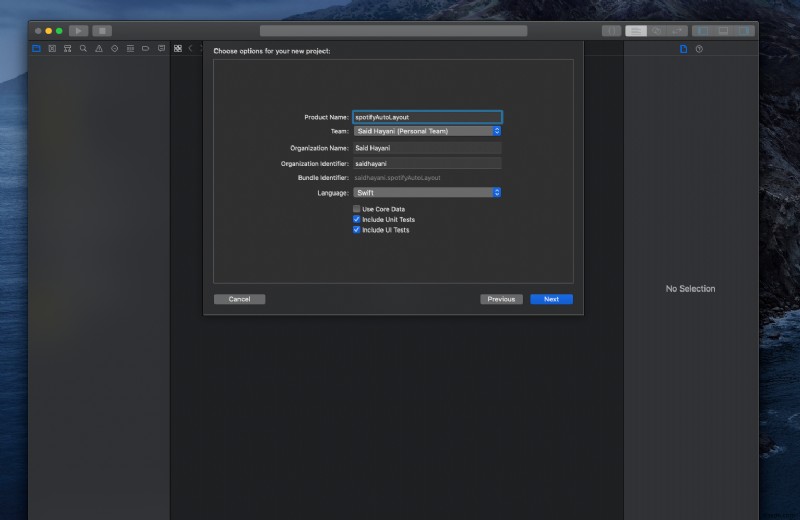
और सबसे पहले हमें ViewController.swift
. में करना होगा सुपरक्लास को UICollectionViewController
. में बदल रहा है UIViewController
. के बजाय क्योंकि हमारी क्लास collectionView
. पर आधारित होगी ।
//
// ViewController.swift
// spotifyAutoLayout
//
// Created by admin on 10/31/19.
// Copyright © 2019 Said Hayani. All rights reserved.
//
import UIKit
class ViewController: UICollectionViewController {
override func viewDidLoad() {
super.viewDidLoad()
collectionView.backgroundColor = .purple
// Do any additional setup after loading the view.
}
}
यदि आप ऐप चलाने का प्रयास करते हैं तो बिल्ड विफल हो जाएगा। हमें AppDelegate.swift
. में कुछ कोड जोड़ने की जरूरत है didFinishLaunchingWithOptions
. के अंदर फ़ाइल करें कोड के इस भाग को return
. से पहले कार्य करें कथन:
let layout = UICollectionViewFlowLayout()
window = UIWindow()
window?.rootViewController = ViewController(collectionViewLayout: layout)
और कोड इस तरह दिखना चाहिए:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
let layout = UICollectionViewFlowLayout()
window = UIWindow()
window?.rootViewController = ViewController(collectionViewLayout: layout)
return true
}
अब आपको ऐप चलाने और backgroundColor
. देखने में सक्षम होना चाहिए purple
. में बदला गया :
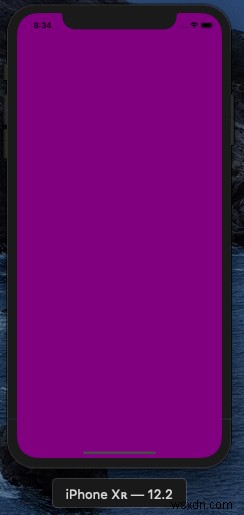
अगला कदम लेआउट को वितरित करना और अनुभागों के बीच समान रूप से स्थान को विभाजित करना है।
आइए हमारे CollectionView
. के तरीकों को परिभाषित करें ।
कदम:
- अद्वितीय पहचानकर्ता के साथ पुन:प्रयोज्य सेल पंजीकृत करें
- अनुभाग में मदों की संख्या निर्धारित करें
- पंजीकृत सेल का उपयोग करें
कुछ CollectionView
. का उपयोग करने के लिए वे तरीके जिन्हें हमें हमेशा UICollectionViewDelegateFlowLayout
. के अनुरूप बनाने की आवश्यकता होती है एक सुपरक्लास के रूप में और विधियों का स्वत:पूर्ण प्राप्त करने के लिए। तो चलिए CollectionViewCell का पंजीकरण शुरू करते हैं।
अंदर View.DidLoad()
हम collectionView.register()
. कहते हैं पुन:प्रयोज्य सेल को पंजीकृत करने की विधि:
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: cellId)
फिर हम collectionView
. के अंदर मौजूद सेल की संख्या को परिभाषित करते हैं numberOfItemsInSection
. का उपयोग करके . अभी के लिए हमें इसे केवल 5 आइटम बनाने की आवश्यकता है:
override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 5
}
अगला चरण cellForItemAt
. का उपयोग करके पुन:प्रयोज्य सेल को परिभाषित करना है जो UICollectionViewCell
return लौटाना चाहिए और उनके पास cellId
. नामक एक अद्वितीय आईडी है . कोड इस तरह दिखता है:
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellId, for: indexPath)
cell.backgroundColor = .red
return cell
}
पूरा कोड इस तरह दिखना चाहिए:
import UIKit
class ViewController: UICollectionViewController, UICollectionViewDelegateFlowLayout {
let cellId : String = "cellId"
override func viewDidLoad() {
super.viewDidLoad()
collectionView.backgroundColor = .purple
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: cellId)
}
override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 5
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellId, for: indexPath)
cell.backgroundColor = .red
return cell
}
}
आपको स्क्रीन पर लाल पृष्ठभूमि वाले 5 आइटम देखने में सक्षम होना चाहिए:
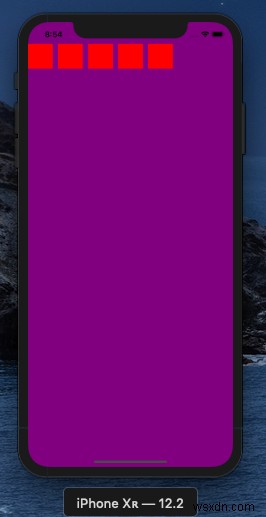
सेल में एक कस्टम चौड़ाई और ऊंचाई जोड़ें
अब हमें कोशिकाओं को सही क्रम में रखना है और उन्हें एक width
देना है और height
. प्रत्येक सेल width
लेगी स्क्रीन के रूप में width
।
हम भाग्यशाली हैं कि हमारे पास sizeForItemAt
है विधि ताकि हम कोशिकाओं को एक कस्टम width
. दे सकें और height
. यह एक ऐसा तरीका है जिसे CGSize
. लौटाना चाहिए टाइप करें:
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
let width = view.frame.width
let height = CGFloat(200)
return CGSize(width: width, height: height)
}
इसलिए हमने Cell
. बनाया width
लें view.frame.width
. का उपयोग करके स्क्रीन का और एक कस्टम height
के साथ एक CGFloat
है टाइप करें।
अब आप अपने सिम्युलेटर में परिणाम नीचे देख सकते हैं:
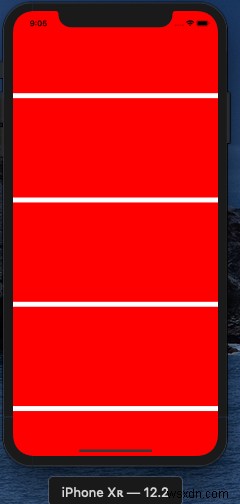
अब तक सब कुछ अच्छा लग रहा है। इस बार एक कस्टम सेल बनाते हैं जिसे पुन:प्रयोज्य किया जा सकता है। CustomCell
. नाम की एक नई स्विफ्ट फ़ाइल बनाएं :
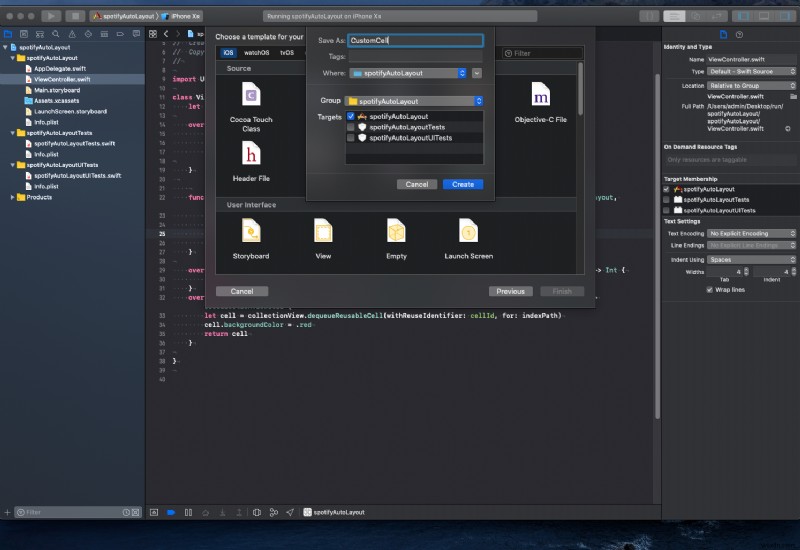
CustomCell.swift
नीचे इस तरह दिखना चाहिए:
import UIKit
class CustomCell: UICollectionViewCell {
override init(frame: CGRect) {
super.init(frame: frame)
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
अब अगली चीज़ जो हमें करनी है वह है पुन:प्रयोज्य सेल का समर्थन करने के लिए दो विधियों को संशोधित करना, collectionView.register
और cellForItemAt
. आइए पहले रजिस्टर विधि को संशोधित करें। UICollectionViewCell.self
बदलें CustomCell
के साथ :
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: cellId)
आगे हमें cellForItemAt
cast डालना होगा CustomCell
. के अनुरूप होना नीचे की तरह:
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellId, for: indexPath) as! CustomCell
यदि आप ऐप चलाते हैं तो शायद आपको कोई बदलाव नज़र नहीं आएगा, इसलिए CustomCell को एक बैकग्राउंड कलर दें backgroundColor = .yellow
. लाइन को हटाना न भूलें cell.backgroundColor = .red
में cellForItemAt
. आपको पृष्ठभूमि का रंग बदलकर पीला देखना चाहिए?
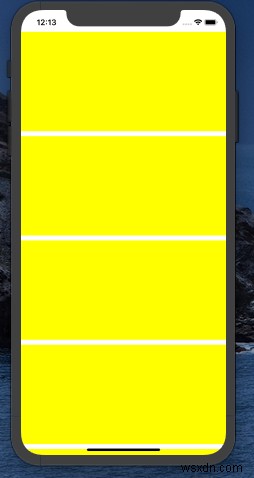
अब CutomCell
. में थोड़ा नमक डालने का समय आ गया है :डी
यदि आप Spotify होम स्क्रीन को देखते हैं, तो प्रत्येक अनुभाग जो एक CustomCell
है हमारे उदाहरण में एक अनुभाग शीर्षक, उप-कोशिकाएँ हैं, और यह क्षैतिज है:

एक अनुभाग शीर्षक जोड़ें
आइए सेल में एक शीर्षक लेबल जोड़ें। titleLabel
बनाएं CutomCell
. के अंदर तत्व कक्षा:
let titleLabel: UILabel = {
let lb = UILabel()
lb.text = "Section Title"
lb.font = UIFont.boldSystemFont(ofSize: 14)
lb.font = UIFont.boldSystemFont(ofSize: 14)
return lb
}()
फिर तत्व को init()
. के अंदर के दृश्य में जोड़ें ब्लॉक करें:
addSubview(titleLabel)
यदि आप ऐप चलाते हैं तो आपको कोई बदलाव नहीं दिखाई देगा, और ऐसा इसलिए है क्योंकि हमने अभी तक तत्व के लिए कोई बाधा नहीं डाली है। तो चलिए कुछ बाधाएँ जोड़ते हैं - यह गुण जोड़ें lb.translatesAutoresizingMaskIntoConstraints = false
करने के लिए titleLabel
तत्व के लिए बाधाओं को लागू करने में सक्षम होने के लिए:
हमारे द्वारा titleLabel
add जोड़ने के बाद देखने के लिए, हम बाधाओं को परिभाषित करते हैं:
addSubview(titleLabel)
titleLabel.topAnchor.constraint(equalTo: topAnchor, constant: 8).isActive = truetitleLabel.leftAnchor.constraint(equalTo: leftAnchor,constant: 8 ).isActive = true
हमेशा .isActive = true
जोड़ना सुनिश्चित करें संपत्ति - इसके बिना बाधा काम नहीं करेगी!
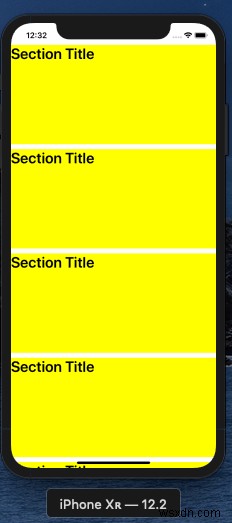
इससे पहले कि हम अगले भाग पर जाएँ, आइए पहले स्क्रीन की पृष्ठभूमि का रंग बदलकर काला करें और सेल के लिए पीला रंग भी हटा दें:
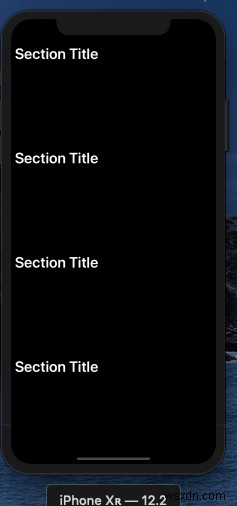
अब बड़ा हिस्सा आता है:प्रत्येक कोशिका में उप-कोशिकाएँ डालना। इसे प्राप्त करने के लिए हम एक CollectionView
जोड़ने जा रहे हैं अंदर CustomCell
।
एक CollectionView
जोड़ने के लिए अंदर UICollectionViewCell
हमें गुण जोड़ने की जरूरत है UICollectionViewDelegate
, UICollectionViewDelegateFlowLayout
, और UICollectionViewDataSource
सुपरक्लास के रूप में CustomCell
।
आइए बनाते हैं collectionView
किसी भी साधारण दृश्य के रूप में तत्व:
let collectionView : UICollectionView = {
// init the layout
let layout = UICollectionViewFlowLayout()
// set the direction to be horizontal
layout.scrollDirection = .horizontal
// the instance of collectionView
let cv = UICollectionView(frame: .zero, collectionViewLayout: layout)
// Activate constaints
cv.translatesAutoresizingMaskIntoConstraints = false
return cv
}()
ध्यान दें कि हम layout
. जोड़ते हैं करने के लिए collectionView
प्रारंभकर्ता में परत के रूप में जैसा कि हमने पहली बार viewController.swift
. के साथ किया था . यहां हम FlowLayout
. की दिशा भी निर्दिष्ट करते हैं होने के लिए .horizontal
।
आइए जोड़ें collectionView
सबव्यू के रूप में देखने के लिए तत्व।
हम एक ऐसा फंक्शन बनाने जा रहे हैं जो हमारे लिए कोड को थोड़ा साफ करने के लिए ऐसा करेगा।
fileprivate func setupSubCells(){
// add collectionView to the view
addSubview(collectionView)
collectionView.dataSource = self
collectionView.delegate = self
// setup constrainst
// make it fit all the space of the CustomCell
collectionView.topAnchor.constraint(equalTo: titleLabel.bottomAnchor).isActive = true
collectionView.leftAnchor.constraint(equalTo: leftAnchor).isActive = true
collectionView.bottomAnchor.constraint(equalTo: bottomAnchor).isActive = true
collectionView.rightAnchor.constraint(equalTo: rightAnchor).isActive = true
}
प्रतिनिधि को self
. पर सेट करना सुनिश्चित करें collectionView
. के लिए और डेटा स्रोत भी:
collectionView.dataSource = self
collectionView.delegate = self
फिर फ़ंक्शन को init
. के भीतर कॉल करें ब्लॉक करें।
यदि आप ऐप बनाने का प्रयास कर रहे हैं तो Xcode कुछ त्रुटियां प्रदर्शित करेगा क्योंकि हम UICollectionViewDelegate
के अनुरूप नहीं हैं और UICollectionViewDelegateFlowLayout
प्रोटोकॉल इसे ठीक करने के लिए हमें सबसे पहले उप सेल को पुन:प्रयोज्य सेल के रूप में पंजीकृत करना होगा।
कक्षा के शीर्ष पर एक चर बनाएँ और इसे cellId
. का नाम दें इसलिए हम इसका उपयोग तब कर सकते हैं जब हमें सेल पहचानकर्ता की आवश्यकता हो:
let cellId : String = "subCellID"
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: cellId)
अब हम त्रुटियों को दूर करने के लिए दो और तरीके खो रहे हैं:numberOfItemsInSection
जो अनुभाग में कक्षों की संख्या को परिभाषित करते हैं और cellForItemAt
जो पुन:प्रयोज्य सेल को परिभाषित करता है। collectionView
. के लिए ये तरीके जरूरी हैं ठीक से काम करने के लिए:
// number of cells
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 4
}
// reusable Cell
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellId, for: indexPath)
cell.backgroundColor = .yellow
return cell
}
परिणाम इस तरह दिखना चाहिए:
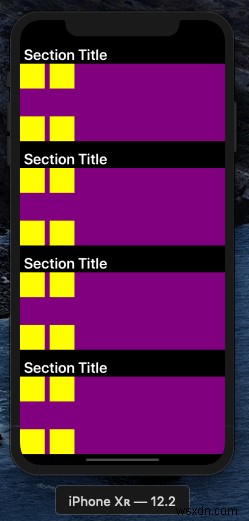
जैसा कि आप देख सकते हैं, collectionView
पृष्ठभूमि के रूप में बैंगनी रंग में हैं और उप-कोशिकाएं पीले रंग की हैं।
इस लेख को समाप्त करने से पहले हम जो आखिरी काम कर सकते हैं, वह है subCells
अनुभाग की ऊंचाई और चौड़ाई के रूप में है। फिर से हम sizeForItemAt
. का उपयोग कर रहे हैं height
को परिभाषित करने के लिए और width
सेल का।
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
let width = frame.height
let height = frame.height
return CGSize(width: width, height: height)
}
और यहाँ हम हैं ?:

अच्छा! मैं इस बिंदु पर रुकने वाला हूं इसलिए यह पोस्ट बहुत लंबी नहीं है। मैं एक दूसरा भाग बनाऊंगा जहां हम कुछ नकली तस्वीरें जोड़ने जा रहे हैं और इसे कुछ डेटा से भर देंगे।
पूर्ण स्रोत कोड? यहाँ
कृपया कृपया यदि आपके पास कोई अतिरिक्त, प्रश्न या सुधार हैं, तो इसे नीचे टिप्पणी में पोस्ट करें? या मुझे ट्विटर पर मारो।
सदस्य बनें इस ट्यूटोरियल का दूसरा भाग प्रकाशित होने पर अधिसूचित होने के लिए मेरी ईमेल सूची में